A Popup PickerView or DatePickerView
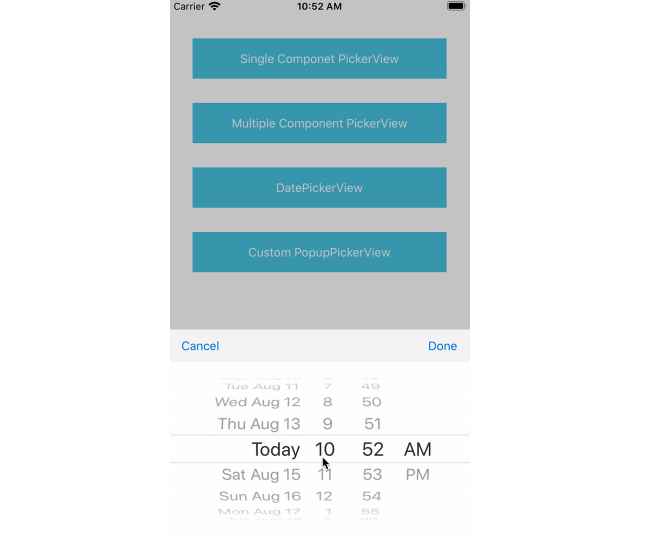
AYPopupPickerView
A Popup PickerView or DatePickerView.
Installation
CocoaPods
pod 'AYPopupPickerView'
Carthage
github "Aiur3908/AYPopupPickerView"
Swift Package Manager
File -> Swift Packages -> Add Package Dependency
https://github.com/Aiur3908/AYPopupPickerView
Manually
Drag and drop Sources folder to your project.
Usage
AYPopupPickerView
let itemsTitle = ["Apple", "Avocado", "Banana", "Cherry", "Coconut", "Grape"]
let popupPickerView = AYPopupPickerView()
popupPickerView.display(itemTitles: itemsTitle, doneHandler: {
let selectedIndex = popupPickerView.pickerView.selectedRow(inComponent: 0)
print(itemsTitle[selectedIndex])
})
AYPopupDatePickerView
let popupDatePickerView = AYPopupDatePickerView()
popupDatePickerView.display(defaultDate: Date(), doneHandler: { date in
print(date)
})
Custom
AYPopupPickerView
public var pickerView: UIPickerView!
public var doneButton: UIButton!
public var cancelButton: UIButton!
public var headerView: UIView!
AYPopupDatePickerView
public var datePickerView: UIDatePicker!
public var doneButton: UIButton!
public var cancelButton: UIButton!
public var headerView: UIView!
Example
let customPopupPickerView = AYPopupPickerView()
customPopupPickerView.headerView.backgroundColor = UIColor.green
customPopupPickerView.doneButton.setTitle("OK", for: .normal)
customPopupPickerView.doneButton.setTitleColor(.red, for: .normal)
customPopupPickerView.cancelButton.setTitleColor(.blue, for: .normal)
let customPopupDatePickerView = AYPopupDatePickerView()
customPopupDatePickerView.datePickerView.datePickerMode = .date
You can implementation UIPickerViewDataSource & UIPickerViewDelegate in your ViewController
class ViewController: UIViewController {
let popupPickerView = AYPopupPickerView()
override func viewDidLoad() {
super.viewDidLoad()
popupPickerView.pickerView.dataSource = self
popupPickerView.pickerView.delegate = self
}
@IBAction func display(_ sender: Any) {
popupPickerView.display(doneHandler: {
})
}
}
extension ViewController: UIPickerViewDataSource & UIPickerViewDelegate {
func numberOfComponents(in pickerView: UIPickerView) -> Int {
return 2
}
func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return 5
}
func pickerView(_ pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String? {
return "\(row)"
}
}
Author
Jerry You
Email: Aiur3908@gmail.com